Sunday, September 29, 2013
Tuesday, February 8, 2011
Understanding WSDL
About WSDL
•WSDL stands for Web Service Definition Language.
•It is a contract to the web service that is expressed in XML. To understand better this can be compared to an Interface in Java or a Package in Oracle which acts as a contract between the client and the system.
Structure of WSDL (parallels are drawn to Java wherever possible)
1.What are the capabilities of the Service?
◦What are the operations(methods) provided by the Service and what are the messages(parameters) that each operation requires?
◦The messages can be input(parameters), output(return value) or faults(exception)
2.How can the Service be accessed?
◦What is the protocol(SOAP) and what is the encoding(document)?
3.Where can the Service be accessed?
◦What is the endpoint(address) of the Service?
Monday, February 7, 2011
Top Down Vs Bottom Up Approach
Wednesday, December 15, 2010
Simple JDBC Program to Access/Read Microsoft Excel
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.Statement;
public class Main {
public static Connection getConnection() throws Exception {
String driver = "sun.jdbc.odbc.JdbcOdbcDriver";
String url = "jdbc:odbc:excelDB";
String username = "yourName";
String password = "yourPass";
Class.forName(driver);
return DriverManager.getConnection(url, username, password);
}
public static void main(String args[]) throws Exception {
Connection conn = null;
Statement stmt = null;
ResultSet rs = null;
conn = getConnection();
stmt = conn.createStatement();
String excelQuery = "select * from [Sheet1$]";
rs = stmt.executeQuery(excelQuery);
while (rs.next()) {
System.out.println(rs.getString("FirstName") + " " + rs.getString("LastName"));
}
rs.close();
stmt.close();
conn.close();
}
}
Read and Write Microsoft Excel ( .xls ) Documents with Jakarta's POI Open source
Reading an existing Excel document is just as simple as creating one. HSSF provides a custom POIFSFileSystem object that specializes in reading Microsoft's OLE 2 documents. Using the POIFSFileSystem object, you construct an HSSFWorkbook object from a specified Excel document. Here's an example.
//create a POIFSFileSystem object to read the data
POIFSFileSystem fs = new POIFSFileSystem(
new FileInputStream(
"c:/dev/src/customs/Book1.xls"));
Next, create a new workbook from the input stream. You can obtain a reference to any of the worksheets within the workbook. Notice that the worksheet index starts at 0.
// create a workbook out of the input stream
HSSFWorkbook wb = new HSSFWorkbook(fs);
// get a reference to the worksheet
HSSFSheet sheet = wb.getSheetAt(0);
A Little Bit More
Some of you may need to use Java to access Excel from a Web application. In such cases, you may need to construct an Excel document and show it in a browser. That's not difficult but does require a little trick. You send the Excel document as the response, but to make it appear in Excel, you need to set the MIME type of the response to the appropriate type. This method is browser independent. Here's an example:
try {
OutputStream out = res.getOutputStream();
// SET THE MIME TYPE
res.setContentType("application/vnd.ms-excel");
// set content dispostion to attachment in
// case the open/save dialog needs to appear
res.setHeader("Content-disposition",
"inline; filename=sample");
workBook.write(out);
out.flush();
out.close();
} catch (FileNotFoundException fne) {
System.out.println("File not found...");
} catch (IOException ioe) {
System.out.println("IOException..." +
ioe.toString());
}
The following points are important:
Use the MIME type string "application/vnd.ms-excel"
In the response header, the content-disposition entry defines how to deliver this content to the browser. For example, an inline attribute specifies that the browser should present an Open/Save/Cancel dialog before opening this document. The filename attribute specifies a default file name for the document.
This tutorial should help you to get started in the world of Java-to-Microsoft bridges. The POI project offers considerably more than I've discussed here, such as the capability to interact with Microsoft Word and with Adobe's PDF files. The project is very active, so you should monitor the POI project and keep up what's going on.
Q : How to read a Microsoft Excel ( .xls ) file from Java?
June 29, 2001
Can I read a Microsoft Excel file from Java? If so, how?
Yes, you can read Microsoft Excel files from Java. Microsoft provides an ODBC driver for Excel, thus allowing you to use JDBC
and the Sun JDBC-ODBC driver to read Excel files.
Assume you have an Excel file named c:\qa.xls
. Also suppose that the data is in a worksheet named qas
and takes the following excel/04-qa-0629-excel-table.html Following format .
Microsoft's ODBC driver treats the first row in a spreadsheet as the column names and the worksheet name as the database
name.
To access a spreadsheet with JDBC, you need to create a new ODBC data source. To create one in Windows 2000:
- Go to "Control Panel"
- Go to "Administrative Tools"
- Go to "Data Sources"
- Select "Add"
- Choose "Microsoft Excel Driver" and "Finish," as seen in Figure 1
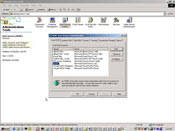
Figure 1. Create new data source
Then give the "Data Source Name" qa-list
and select the workbook, shown in Figure 2.
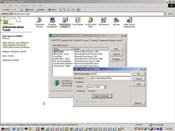
Figure 2. ODBC Microsoft Excel Setup
When you are done you should see your new qa-list
data source name:
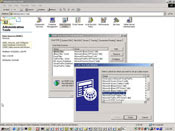
Figure 3. New listing of user data sources
Now the spreadsheet is available as an ODBC source.
Say that you would like to pull out all March 2000 entries. You will need to hit the data source with the following SQL query:
select URL from [qas$] where Month='March' and Year=2000;
Note that the table name is the name of the worksheet with a $
appended to the end. You have to append the $
in order for the query to work. Why? Because. The brackets are there because $
is a reserved character in SQL. Life is never easy.
Now try ExcelReader
on for size:
import java.sql.Connection;
import java.sql.Statement;
import java.sql.ResultSet;
import java.sql.DriverManager;
public class ExcelReader
{
public static void main( String [] args )
{
Connection c = null;
Statement stmnt = null;
try
{
Class.forName( "sun.jdbc.odbc.JdbcOdbcDriver" );
c = DriverManager.getConnection( "jdbc:odbc:qa-list", "", "" );
stmnt = c.createStatement();
String query = "select URL from [qas$] where Month='March' and Year=2000;";
ResultSet rs = stmnt.executeQuery( query );
System.out.println( "Found the following URLs for March 2000:" );
while( rs.next() )
{
System.out.println( rs.getString( "URL" ) );
}
}
catch( Exception e )
{
System.err.println( e );
}
finally
{
try
{
stmnt.close();
c.close();
}
catch( Exception e )
{
System.err.println( e );
}
}
}
}
In ExcelReader
, the main()
gets a connection to the spreadsheet, then pulls out all of the entries for March 2000.
Saturday, November 15, 2008
10- 20 C questions
{
char string[]="Hello World";
display(string);
}
void display(char *string)
{
printf("%s",string);
}
Answer:
Compiler Error : Type mismatch in redeclaration of function display
Explanation :
In third line, when the function display is encountered, the
compiler doesn't know anything about the function display. It assumes the
arguments and return types to be integers, (which is the default type). When it
sees the actual function display, the arguments and type contradicts with what it
has assumed previously. Hence a compile time error occurs.
12. main()
{
int c=- -2;
printf("c=%d",c);
}
Answer:
c=2;
Explanation:
Here unary minus (or negation) operator is used twice. Same
maths rules applies, ie. minus * minus= plus.
Note:
However you cannot give like --2. Because -- operator can only be
applied to variables as a decrement operator (eg., i--). 2 is a constant and not a
variable.
13. #define int char
main()
{
int i=65;
printf("sizeof(i)=%d",sizeof(i));
}
Answer:
sizeof(i)=1
Explanation:
Since the #define replaces the string int by the macro char
14. main()
{
int i=10;
i=!i>14;
Printf ("i=%d",i);
}
Answer:
i=0
Explanation:
In the expression !i>14 , NOT (!) operator has more precedence
than ‘ >’ symbol. ! is a unary logical operator. !i (!10) is 0 (not of true is false).
0>14 is false (zero).
15. #include
main()
{
char s[]={'a','b','c','\n','c','\0'};
char *p,*str,*str1;
p=&s[3];
str=p;
str1=s;
printf("%d",++*p + ++*str1-32);
}
Answer:
77
Explanation:
p is pointing to character '\n'. str1 is pointing to character 'a' ++*p. "p is
pointing to '\n' and that is incremented by one." the ASCII value of '\n' is 10,
which is then incremented to 11. The value of ++*p is 11. ++*str1, str1 is
pointing to 'a' that is incremented by 1 and it becomes 'b'. ASCII value of 'b' is
98.
Now performing (11 + 98 – 32), we get 77("M");
So we get the output 77 :: "M" (Ascii is 77).
16. #include
main()
{
int a[2][2][2] = { {10,2,3,4}, {5,6,7,8} };
int *p,*q;
p=&a[2][2][2];
*q=***a;
printf("%d----%d",*p,*q);
}
Answer:
SomeGarbageValue---1
Explanation:
p=&a[2][2][2] you declare only two 2D arrays, but you are trying
to access the third 2D(which you are not declared) it will print garbage values.
*q=***a starting address of a is assigned integer pointer. Now q is pointing to
starting address of a. If you print *q, it will print first element of 3D array.
17. #include
main()
{
struct xx
{
int x=3;
char name[]="hello";
};
struct xx *s;
printf("%d",s->x);
printf("%s",s->name);
}
Answer:
Compiler Error
Explanation:
You should not initialize variables in declaration
18. #include
main()
{
struct xx
{
int x;
struct yy
{
char s;
struct xx *p;
};
struct yy *q;
};
}
Answer:
Compiler Error
Explanation:
The structure yy is nested within structure xx. Hence, the elements
are of yy are to be accessed through the instance of structure xx, which needs an
instance of yy to be known. If the instance is created after defining the structure
the compiler will not know about the instance relative to xx. Hence for nested
structure yy you have to declare member.
19. main()
{
printf("\nab");
printf("\bsi");
printf("\rha");
}
Answer:
hai
Explanation:
\n - newline
\b - backspace
\r - linefeed
20. main()
{
int i=5;
printf("%d%d%d%d%d%d",i++,i--,++i,--i,i);
}
Answer:
45545
Explanation:
The arguments in a function call are pushed into the stack from left
to right. The evaluation is by popping out from the stack. and the evaluation is
from right to left, hence the result.
21. #define square(x) x*x
main()
{
int i;
i = 64/square(4);
printf("%d",i);
}
Answer:
64
Explanation:
the macro call square(4) will substituted by 4*4 so the expression
becomes i = 64/4*4 . Since / and * has equal priority the expression will be
evaluated as (64/4)*4 i.e. 16*4 = 64
22. main()
{
char *p="hai friends",*p1;
p1=p;
while(*p!='\0') ++*p++;
printf("%s %s",p,p1);
}
Answer:
ibj!gsjfoet
Explanation:
++*p++ will be parse in the given order
*p that is value at the location currently pointed by p will be taken
++*p the retrieved value will be incremented
when ; is encountered the location will be incremented that is p++ will be
executed
Hence, in the while loop initial value pointed by p is ‘h’, which is changed to ‘i’ by
executing ++*p and pointer moves to point, ‘a’ which is similarly changed to ‘b’
and so on. Similarly blank space is converted to ‘!’. Thus, we obtain value in p
becomes “ibj!gsjfoet” and since p reaches ‘\0’ and p1 points to p thus p1doesnot
print anything.